Bitwise Right shift >> operator
Bitwise Right shift operator >> is used to shift the binary sequence to right side by specified position.
Example
Let’s take a number 14.
Binary representation of 14 is 00001110 (for the sake of clarity let’s write it using 8 bit)
14 = (00001110) 2
Then 14 >> 1 will shift the binary sequence by 1 position to the right side.
Like,
Pictorial Explanation
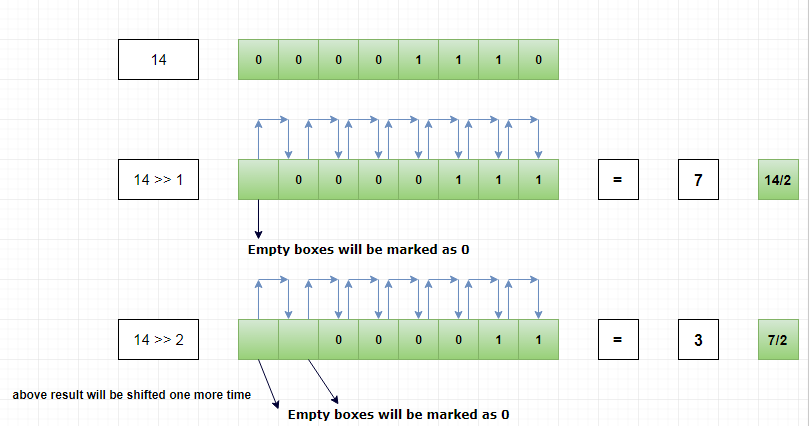
Application of Bitwise Right Shift Operator
In the above diagram, you can notice that whenever we shift the number one position to right, the output value will be exactly number / 2.
If I shift 14 by 1 position to the right, output will be 14 / 2 = 7. i.e 14/2 = 7
If I shift 14 by 2 position to the right, output will be 14 / 4 = 3. i.e 14/4 =3.5 since it’s an integer, fractional part will not be considered.
In general, if we shift a number by n times to right, the output will be number / (2n) .
Example
Let’s assume number as 128.
If we shift the number 5 position to the right, the output will be
= 128 >> 5
= 128 / (25)
=128/32
=4.
Program using bitwise right shift operator
Example
#include<stdio.h> int main() { int var = 128; printf("var/2 =%d \n",var>>1); //1 position to right printf("var/4 =%d \n",var>>2); //2 position to right printf("var/8 =%d \n",var>>3); //3 position to right printf("var/16 =%d \n",var>>4); //4 position to right printf("var/32 =%d \n",var>>5); //5 position to right return 0; }