Accessing string using pointer
Using char* (character pointer), we can access the string.
Example
Declare a char pointer
Assign the string base address(starting address) to the char pointer.
char str[6] = "Hello"; char *ptr; //string name itself base address of the string ptr = str; //ptr references str
Where,
ptr - is a character pointer which points the first character of the string. i.e. &str[0]
Like normal pointer arithmetic, if we move the ptr by 1 (ptr+1) position it will point the next character.
Printing each character address of a string using pointer variable
Example
/* * Program : printing address of each char in the string * using pointer variable * Language : C */ #include<stdio.h> int main() { char str[6] = "Hello"; char *ptr; int i; //string name itself a base address of the string ptr = str; //ptr references str for(i = 0; ptr[i] != '\0'; i++) printf("&str[%d] = %p\n",i,ptr+i); return 0; }
Printing each character of a string using the pointer
To print the value stored at each char address, we have to dereference the memory address.
Like below,
*(ptr+i)
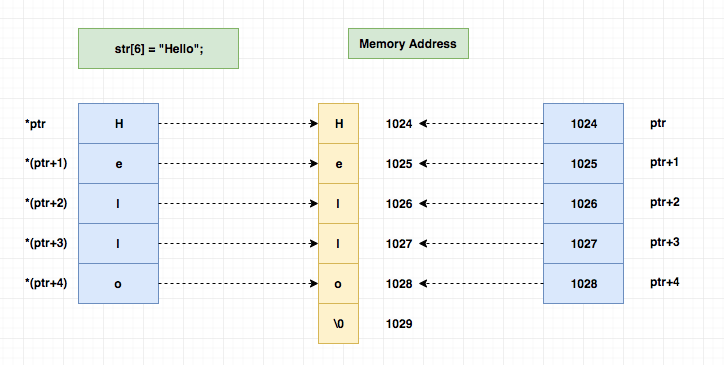
Example
/* * Program : printing each char in the string * using pointer variable * Language : C */ #include<stdio.h> int main() { char str[6] = "Hello"; char *ptr; int i; //string name itself a base address of the string ptr = str; //ptr references str for(i = 0; ptr[i] != '\0'; i++) printf("str[%d] = %c\n",i,*(ptr+i)); return 0; }
Manipulating characters of a string using the pointer
Let's change the 4th(index 3) character 'l' as 'o'.
The new string will be "Heloo".
Example
/* * Program : Manipulating string using pointer * Language : C */ #include<stdio.h> int main() { char str[6] = "Hello"; char *ptr; int i; printf("String = %s\n", str); //string name itself a base address of the string ptr = str; //ptr references str //change the 4th char as 'o' *(ptr+3) = 'o'; printf("Updated string = %s\n",str); return 0; }