Get string input from user and print it
Using %s format specifier in scanf, we can get string input from the user.
Example
char name[20]; scanf("%s",name);
Note
In scanf we didn’t give &name. Why?
Because string name itself base address of a string.
Pictorial Explanation
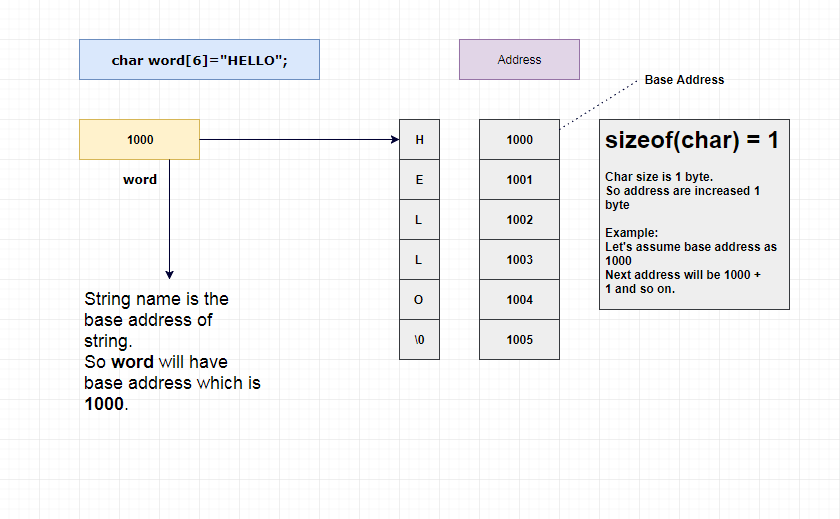
So, no need to give & operator in scanf while getting string input.
%s in printf
If we give %s to print a string,
It will print every character of a string one by one until it encounters null character ‘\0’.
If it hits the null character, it will stop printing characters.
So, null character is very important in string. Otherwise, it will keep on print data which may show some sensitive data to attackers.
Sample Program
Example
#include<stdio.h> int main() { char name[10]; printf("Enter your name\n"); //get string input. Note that We are not using &name here. scanf("%s",name); //print the name printf("Welcome %s\n",name); return 0; }
Output
Enter your name
Computer
Welcome Computer