Check whether the given number is Palindrome or Not
Palindrome numbers will remain the same when it’s digits are reversed.
Like, 99,121,323,505 etc
Example
Case 1
num = 121
after digits’ reversal
reverse of num = 121
Output:
Palindrome (121 == 121)
Case 2
num = 345
after digits’ reversal
reverse of num = 543
Output:
Not Palindrome (345 != 543)
Logic
Previous tutorial, we know how to reverse a number
Just apply the concept here.
Get a number from user
Reverse the number
If both number and reversed number are equal
     Print “Palindrome Number”
Else
     Print “Non-Palindrome Number”
Important thing to note in reversing a number is, after the loop execution the value of num becomes zero.
Because each time we are dividing num by 10.
Pictorial Explanation
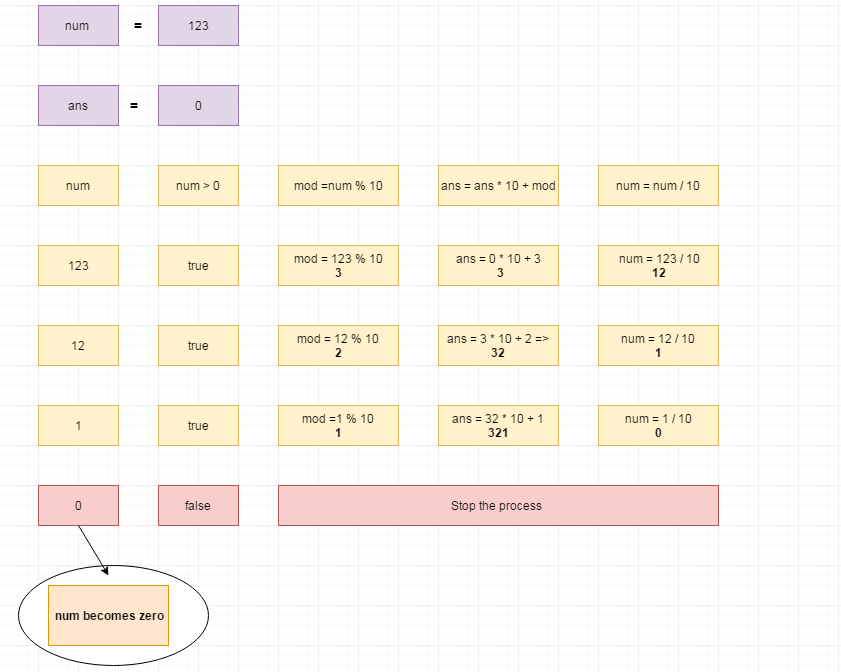
So, num will be always zero after the process. So, we have to copy the num value to another variable for further checking.
Palindrome Number
num = 121
Pictorial Explanation
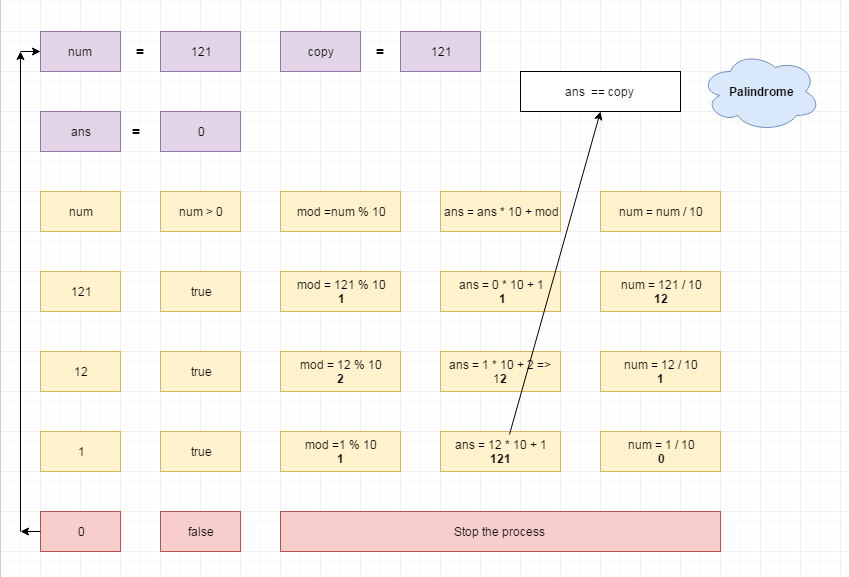
Non-Palindrome Number
num = 123
Pictorial Explanation
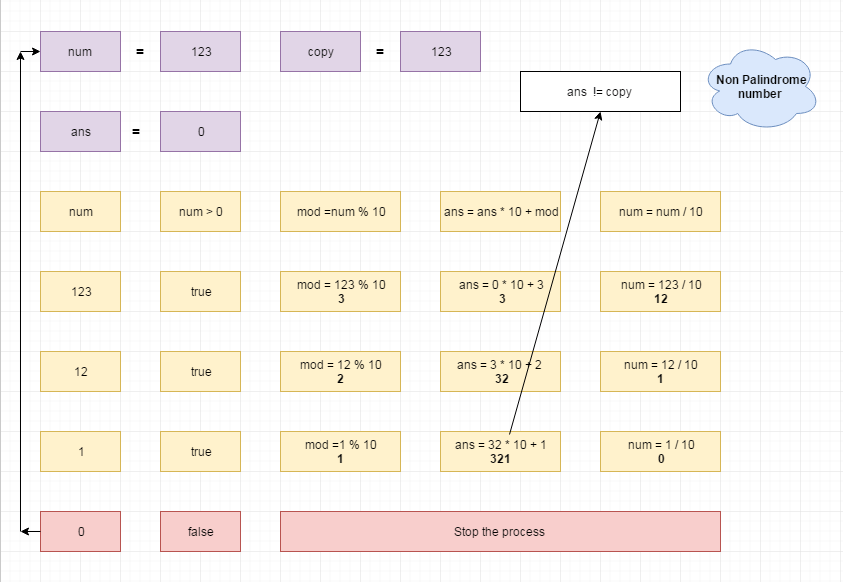
Program
Example
#include<stdio.h> int main() { //variable ans will have the reversed number int num, ans = 0,copy; //get input from user scanf("%d",&num); //assigning num value to variable copy for checking //Because after while loop num value becomes zero. copy = num; //do till num > 0 while(num > 0) { //split the last digit int mod = num % 10; //multiply ans with 10 and add the splitted digit ans = ans * 10 + mod; //divide num by 10 num = num / 10; } //if both reversed and original number are equal, print palindrome if(ans == copy) printf("Palindrome\n"); else printf("Non-Palindrome\n"); //otherwise print non-palindrome return 0; }