Split a number into digits
Get a positive number from user and print each digits of a number.
Example 1
num = 12
output
2
1
Example 2
num = 123
output
3
2
1
Let’s design a logic
num = 12
First let us think, how to split the last digit 2 from the number 12.
Since the numbers are decimal (base 10), each digit’s range should be 0 to 9.
So, we can get it easy by doing modulo 10.
Like,
12 % 10 => 2 ( we have split the digit 2 from number 12)
Now, we have to split the digit 1 from number 12.
This can be achieved by dividing the number by 10 and take the modulo 10.
Like,
12 / 10 => 1
Now take modulo 10
1 % 10 = 1
Using above method, we can split each digit from a number.
Logic
Till number becomes 0
do
   Take number % 10 and print the result
   Divide the number by 10. (number = number / 10)
Example
Let’s take num = 123
Step 1
Num = 123 (not equal to zero)
Mod = 123 % 10 ==> 3
Num = 123 / 10 ==> 12
Step 2
Num = 12 (not equal to 0)
Mod = 12 % 10 ==> 2
Num = 12 / 10 ==> 1
Step 3
Num = 1 (not equal to 0)
Mod = 1 % 10 ==> 1
Num = 1 / 10 ==> 0
Step 4
Num = 0 (equal to zero, stop the process)
Pictorial Explanation
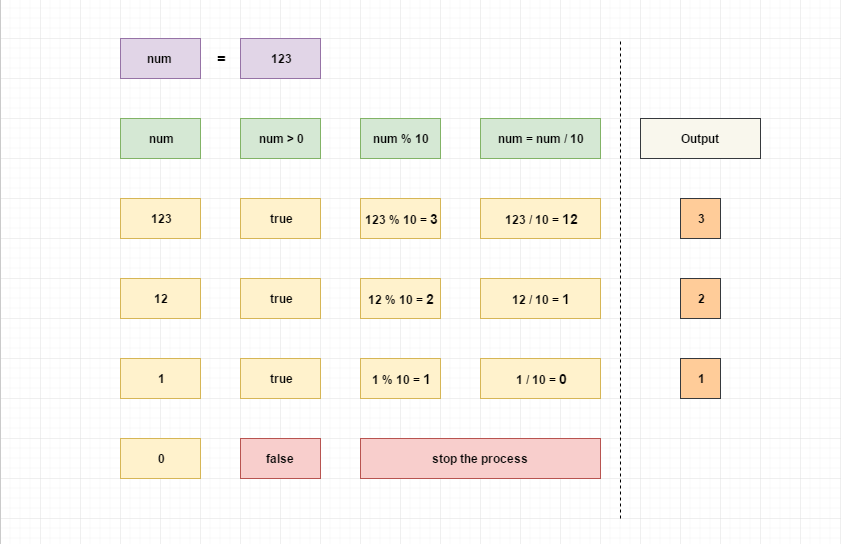
Program
Example
/***************************** *Program : split the digits * *Language : C * *****************************/ #include<stdio.h> int main() { int num; scanf("%d",&num); while(num > 0) //do till num greater than 0 { int mod = num % 10; //split last digit from number printf("%d\n",mod); //print the digit. num = num / 10; //divide num by 10. num /= 10 also a valid one } return 0; }