Bitwise Left shift << operator
Bitwise Left shift operator is used to shift the binary sequence to the left side by specified position.
Example
Let’s take a number 14.
Binary representation of 14 is 00001110 (for the sake of clarity let’s write it using 8 bit)
14 = (00001110) 2
Then 14 << 1 will shift the binary sequence 1 position to the left side.
Like,
Pictorial Explanation
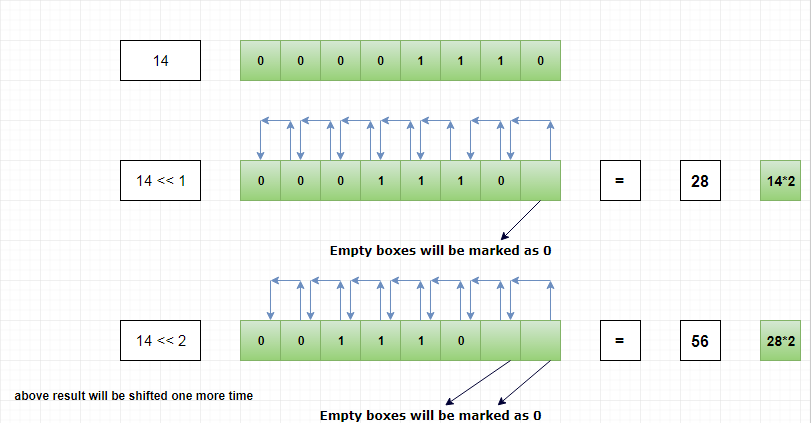
Application of Bitwise Left Shift Operator
In the above diagram, you can notice that whenever we shift the number one position to left, the output value will be exactly number * 2.
If we shift 14 by 1 position to the left, output will be 14 * 2 = 28.
If we shift 14 by 2 position to the left, output will be 14 * 4 = 56.
In general, if we shift a number by n position to left, the output will be number * (2n).
Example
Let’s assume the number as 12
If we shift the number 3 position to the left.
Then the output will be,
Example
12 << 3
= 12 * (23)
= 12 * 8
= 96.
Program using Bitwise Left shift Operator
Example
#Bitwise left shift operator in python var = 2; print("var = ", var<<0) #same number print("var * 2 = ", var<<1) #1 position to the left print("var * 4 = ", var<<2) #2 position to the left print("var * 8 = ", var<<3) #3 position to the left print("var * 16 = ", var<<4) #4 position to the left print("var * 32 = ", var<<5) #5 position to the left